Smart contracts are essential to how blockchain technology operates, acting as self-executing programs that perform tasks automatically when specific conditions are met. However, smart contract vulnerabilities can lead to serious issues, including the loss of millions of dollars, often due to small errors in their code. In this blog, we’ll explore the most common vulnerabilities in smart contracts and explain how they can be addressed in simple terms.
1.Reentrancy Attacks
What is it?
A reentrancy attack is a common vulnerability in smart contracts that can have catastrophic consequences. This attack occurs when a malicious contract exploits the ability to repeatedly call a function in another contract before the previous execution is completed. By doing this, the attacker can manipulate the state of the targeted contract, often leading to significant loss of funds.
Imagine a system where you can withdraw money from your account. Instead of waiting for the system to update your balance after one withdrawal, you find a loophole that lets you make multiple withdrawals before the system realizes the funds are gone. This is essentially how a reentrancy attack works in the context of smart contracts.
Example:
One of the most infamous cases of a reentrancy attack was the DAO (Decentralized Autonomous Organization) hack in 2016. The DAO was a groundbreaking project that allowed users to pool funds and vote on investment proposals. However, its smart contract had a reentrancy vulnerability.
Here’s what happened:
- An attacker exploited the withdrawal function in the DAO contract.
- Instead of updating the user’s balance after each withdrawal, the function allowed repeated calls before the contract could update its state.
- The attacker siphoned off approximately $60 million worth of Ether, causing widespread panic and eventually leading to a controversial hard fork in the Ethereum blockchain.
Fix:
Follow the “checks-effects-interactions” approach: First, complete all state updates in the contract before initiating any external calls.
Add a reentrancy guard, such as Open Zeppelin’s Reentrancy Guard in Solidity.
2.Integer Overflow and Underflow
What is it?
Integer overflow and underflow are common vulnerabilities in smart contracts that occur when arithmetic operations exceed or fall below the limits of a data type. These errors can result in unexpected behavior, leading to serious security issues.
Integer Overflow: This happens when a number exceeds the maximum value allowed by its data type and wraps around to the minimum value.
Integer Underflow: This occurs when a number goes below the minimum value allowed by its data type and wraps around to the maximum value.
Example:
2018 Ethereum Token Exploits:
Several Ethereum-based tokens were exploited due to integer overflow and underflow vulnerabilities. Attackers manipulated token balances, effectively creating unlimited tokens. This led to significant losses and eroded trust in affected projects.
BatchOverflow Vulnerability (2018):
A vulnerability in the “BatchOverflow” smart contract allowed attackers to generate billions of tokens out of thin air, impacting multiple ERC-20 tokens.
Learn more about integer overflow and underflow.
Fix:
1.Use Safe Math Libraries:
Libraries like OpenZeppelin’s SafeMath
ensure that arithmetic operations are performed safely. If an operation causes an overflow or underflow, the transaction reverts. Explore Safe Math here.
2.Leverage Solidity 0.8.0 or Newer:
Solidity 0.8.0 introduced built-in checks for arithmetic operations. By default, operations revert when an overflow or underflow occurs, eliminating the need for external libraries in most cases.
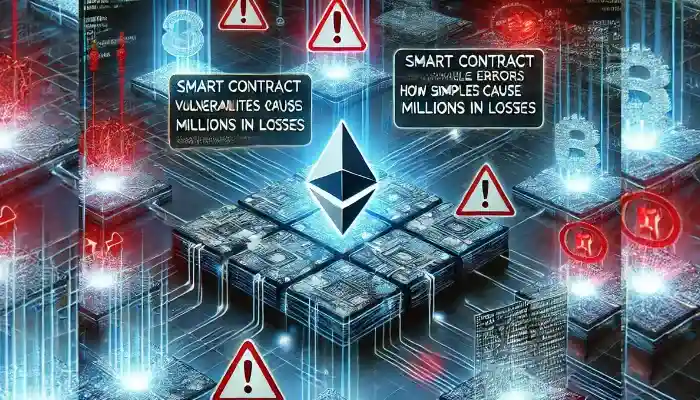
3.Unchecked External Calls
What is it?
Smart contracts often interact with other contracts or external accounts to execute specific operations. However, if these external calls are not carefully checked, they can fail silently or be exploited by malicious actors, leading to financial losses or unexpected behavior.
In essence, an unchecked external call is a call to another contract where the return value is not verified. This oversight can leave the contract vulnerable to exploits or unpredictable outcomes.
Example:
One notable example is the Parity Wallet Hack (2017):
An attacker exploited a vulnerability in the wallet’s external call logic, leading to the accidental freezing of $150 million worth of Ether. This incident highlighted the critical importance of carefully handling external calls.
Fix:
1.Verify Return Values:
Always check the return value of an external call to ensure it has executed successfully. For example, use Solidity’s require
statement to halt execution if the call fails.
2.Use Low-Level Calls Sparingly:
Avoid using low-level calls (e.g., call
, delegatecall
) unless absolutely necessary. These calls bypass Solidity’s built-in checks and are more prone to errors.
4.Timestamp Dependence
What is it?
Timestamp dependence is a vulnerability in smart contract logic where the contract relies on block timestamps for critical operations. While timestamps are a convenient way to measure time on the blockchain, they are not entirely reliable because miners have the ability to manipulate them within a certain range.
In essence, if a smart contract’s functionality depends on the exact value of a block timestamp, it can become vulnerable to exploitation. Miners can slightly adjust timestamps to their advantage, which may lead to unintended behavior in the contract.
Real-Life Example: Vulnerabilities in Lottery Contracts
In early Ethereum smart contracts, several lottery systems relied on block timestamps to determine winners. Attackers exploited this by mining blocks with adjusted timestamps, allowing them to influence the outcome and claim the rewards. This highlighted the need for more secure and tamper-resistant mechanisms.
Fix:
1.Avoid Using Block Timestamps for Critical Logic:
Do not rely on block timestamps for determining outcomes in applications like lotteries, deadlines, or pricing mechanisms. Use alternative methods where possible.
2.Use Block Numbers for Time Calculations:
Instead of using timestamps, calculate time intervals based on block numbers. Since the average block time on Ethereum is relatively stable, this method provides a more predictable and tamper-resistant way to measure time. Read more on time-dependent logic in smart contracts.
5.Lack of Access Control
What is it?
Access control is a fundamental aspect of smart contract security, ensuring that only authorized users can execute specific functions. A lack of access control means that anyone can call these functions, potentially leading to unauthorized actions, financial losses, or a complete compromise of the contract.
Smart contracts without robust access control mechanisms are particularly vulnerable, as malicious actors can exploit this oversight to manipulate the contract’s behavior, steal funds, or cause irreparable damage.
Example:
One of the most infamous examples of inadequate access control occurred in 2017 with the Parity Wallet. A vulnerability in the contract allowed an attacker to reinitialize the contract’s ownership and subsequently lock $150 million worth of Ether.
How it Happened:
- The wallet contract had a function intended for initialization, but it lacked proper access restrictions.
- An attacker called this function, claiming ownership of the contract.
- Once in control, the attacker locked the funds, rendering them inaccessible to legitimate users.
This incident underscored the catastrophic consequences of failing to implement proper access control mechanisms.
Fix:
1.Use “onlyOwner” Modifiers:
Use access control modifiers like onlyOwner
to restrict sensitive functions to the contract owner.
2.Implement Role-Based Access Control (RBAC):
- For more complex contracts, use role-based access control systems to assign specific permissions to different users.
- Libraries like OpenZeppelin’s
AccessControl
make implementing RBAC straightforward.
Conclusion
Smart contracts are undoubtedly powerful tools; however, they come with risks if not properly secured. Therefore, by understanding these vulnerabilities and consistently applying the recommended fixes, developers can create much safer contracts and effectively prevent costly errors. Moreover, following Ethereum’s security best practices further strengthens the reliability of your smart contracts
Related Posts:-
Solana Congestion Problem: Fixing the Network That Stopped Working
Your article helped me a lot, is there any more related content? Thanks!