Running into a “CUDA Out of Memory” error while fine-tuning your large language model on PyTorch? Don’t worry, it is a common and fixable problem. The fastest way to resolve this issue is to reduce your training batch size, enable mixed precision (FP16 or BF16), and turn on gradient checkpointing. If you are using Hugging Face Transformers, you can also offload parts of the model to the CPU or apply 4-bit quantization with tools like bitsandbytes. These fixes significantly reduce memory usage, allowing you to train large models even on limited VRAM (like 8GB or 12GB GPUs). If one fix does not work alone, try combining two or more – for example, using both mixed precision and gradient checkpointing together.
Why This Happens?
When fine-tuning large models like LLaMA, GPT, or BERT on a local GPU, the model can demand more memory than your GPU has available. PyTorch then throws the dreaded CUDA out-of-memory error. It’s like trying to fit a gallon of data into a pint-sized GPU — it simply overflows.
Even high-end GPUs (e.g., 24GB VRAM) can struggle, especially when dealing with long input sequences, large batch sizes, or full-precision (FP32) weights. But don’t worry — you don’t always need a GPU upgrade. You just need to tune your training process smartly.
Step-by-Step Fixes
Here’s how to fix the issue step-by-step:
1. Reduce the Batch Size:
# Example
trainer = Trainer(
train_batch_size=1, # Try setting to 1 or 2
...
)
Smaller batches reduce memory usage, though they might make training slower.
2. Use Gradient Accumulation:
Simulate larger batch sizes without actually using more memory.
# Example
gradient_accumulation_steps = 4 # Effective batch size = batch_size * accumulation
3. Enable Gradient Checkpointing:
This reduces memory by recomputing some layers during backpropagation.
model.gradient_checkpointing_enable()
Add this after loading your model.
4. Use FP16 or BF16 (Mixed Precision Training):
Saves a lot of memory by using half-precision floats.
from transformers import TrainingArguments
training_args = TrainingArguments(
fp16=True, # Or bf16=True if your GPU supports BF16
)
5. Offload to CPU or Use Accelerate:
If you’re using Transformers, use accelerate
to offload weights:
accelerate config
accelerate launch train.py
Use cpu_offload=True
In your training loop to shift some computations to the CPU.
6. Model Quantization:
Convert model weights to lower precision (like INT8 or 4-bit) using libraries like BitsAndBytes.
from transformers import AutoModelForCausalLM, BitsAndBytesConfig
bnb_config = BitsAndBytesConfig(load_in_4bit=True)
model = AutoModelForCausalLM.from_pretrained("model", quantization_config=bnb_config)
7. Clear Cache & Use torch.no_grad()
During Validation:
import torch
torch.cuda.empty_cache()
Also, avoid storing intermediate variables that require gradients when they aren’t needed.
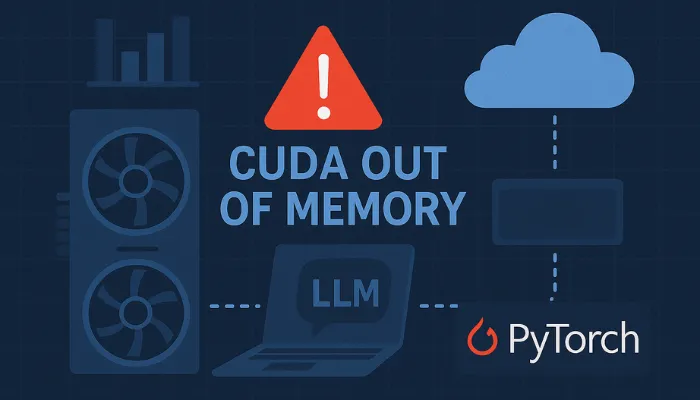
Final Tips and Warnings
These aren’t just “nice-to-know” — they are crucial habits that can save your sanity when working with large models on limited hardware.
1. Monitor VRAM Usage:
Before you even hit “Train,” open your terminal and run:
watch -n 0.5 nvidia-smi
This shows you how much GPU memory is being used in real time, how many processes are active, and whether memory is being released after each epoch. If you notice memory not freeing up after your training stops, that’s a sign of a memory leak or a leftover variable still referencing GPU tensors.
Pro tip: Use this together with top or htop to monitor CPU and RAM as well.
2. Trim Unnecessarily Long Input Sequences:
Transformer models like BERT, GPT, and LLaMA have memory complexity that grows quadratically with input length. That means going from 512 tokens to 1024 tokens could more than double your memory usage.
Even if your raw dataset has long text, consider whether the task really needs that much context. Often, a well-trimmed 256 or 512-token input performs just as well, and keeps your training from crashing.
inputs = tokenizer(text, max_length=512, truncation=True, padding='max_length')
3. Restart Your Kernel Occasionally:
In environments like Jupyter Notebooks or Google Colab, running multiple cells repeatedly can leave variables in memory, even if you reassign them. PyTorch might not release memory back to the system unless the entire process is restarted.
So, if you’ve been tweaking code for a while and start getting unexpected CUDA out-of-memory errors, even when doing less work, try a kernel restart.
4. Don’t Use model.eval()
During Training:
This is a common misunderstanding: model.eval() does not conserve memory. Instead, it changes the behavior of layers like dropout and batch normalization, which are essential for model generalization during training.
Using .eval() during training can silently harm your model’s performance. Only use it when:
- Evaluating on a validation set
- Running inference
- Exporting the model
Remember: Memory-saving strategies should come from techniques like gradient checkpointing or mixed precision, not by disabling training behaviors.
Bonus Tip: Clear Cache Only When Needed
While it’s tempting to add torch.cuda.empty_cache() everywhere, it doesn’t free up memory held by tensors still in scope — it only releases memory marked as unused by PyTorch. Use it after catching OOM errors or before a new training phase, but avoid using it inside tight training loops.
Did This Solve Your Issue?
Try these solutions and let us know which one worked best for you! Fine-tuning LLMs can feel heavy, but with the right tweaks, you can make it GPU-friendly without sacrificing too much performance.
You can also visit:- How to Build a Private AI Model Using Open-Source LLMs